Hello Guys, here i am explaining about how can we play video as layout background in place of image. There are two ways to do this
We can achieve this functionality by VideoView, but video layout can be it may be cut in different devices because VideoView offers Media Controller panel for play-pause video we can hide this panel but video can not play in full view.
On other side SurfaceView basically do the same functionality without any design break. So in our case we use SurfaceView.
Let's do step by step
First of all create folder "raw" in res directory in project structure and place your mp4 video file in it. Make sure video is in potrait mode so it can fit to screen well.
STEP : 1 Create layout.xml
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/coordinatorLayout" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.chirag.videoviewdemoonsplash.LoginActivity" tools:layout_editor_absoluteY="25dp"> <SurfaceView android:id="@+id/videoView" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/email_sign_in_button" style="?android:textAppearanceSmall" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="511dp" android:background="@color/colorPrimary" android:drawableLeft="@android:drawable/ic_lock_idle_lock" android:drawablePadding="10dp" android:paddingLeft="50dp" android:paddingRight="50dp" android:text="Sign In" android:textColor="#FFF" android:textStyle="bold" app:layout_constraintBottom_toTopOf="@+id/textview_or" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/email_sign_up_button" style="?android:textAppearanceSmall" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="17dp" android:background="@color/colorPrimary" android:drawableLeft="@android:drawable/ic_menu_edit" android:drawablePadding="10dp" android:drawableTint="@color/colorWhite" android:drawableTintMode="src_in" android:paddingLeft="45dp" android:paddingRight="45dp" android:text="Sign Up" android:textColor="#FFF" android:textStyle="bold" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toBottomOf="@+id/textview_or" /> <TextView android:id="@+id/textview_or" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:text="OR" android:textColor="@color/colorWhite" app:layout_constraintBottom_toTopOf="@+id/email_sign_up_button" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toBottomOf="@+id/email_sign_in_button" /> </android.support.constraint.ConstraintLayout>
STEP : 2 Create new style for full screen view in style.xml
<!-- Base application theme. --> <style name="SplashTheme" parent="Theme.AppCompat.Light.NoActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">#000</item> <item name="colorAccent">#FFF</item> <item name="windowActionBar">false</item> <item name="windowNoTitle">true</item> </style>
Use this style in AndroidMenifiest.xml in tag
<activity android:name=".LoginActivity" android:theme="@style/SplashTheme" android:label="@string/app_name" android:windowSoftInputMode="adjustPan|adjustResize" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
STEP 3: Finally create LoginActivity.java
public class LoginActivity extends AppCompatActivity { private SurfaceView videoView; private MediaPlayer mediaPlayer; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); // Set up the login form. videoView = findViewById(R.id.videoView); startVideoPlayBack(); } private void startVideoPlayBack() { try { mediaPlayer = MediaPlayer.create(this, R.raw.startup); SurfaceHolder holder = videoView.getHolder(); holder.addCallback(new SurfaceHolder.Callback() { @Override public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) { } @Override public void surfaceCreated(SurfaceHolder holder) { mediaPlayer.setDisplay(holder); mediaPlayer.start(); mediaPlayer.setLooping(true); } @Override public void surfaceDestroyed(SurfaceHolder holder) { } }); } catch (Throwable e) { e.printStackTrace(); } } }
OUTPUT
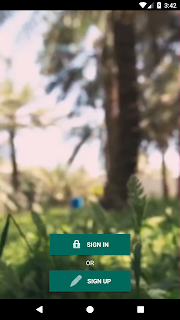
Comments
Post a Comment
Thanks, I'll respond you soon!